How to create your own YouTube video downloader in python?
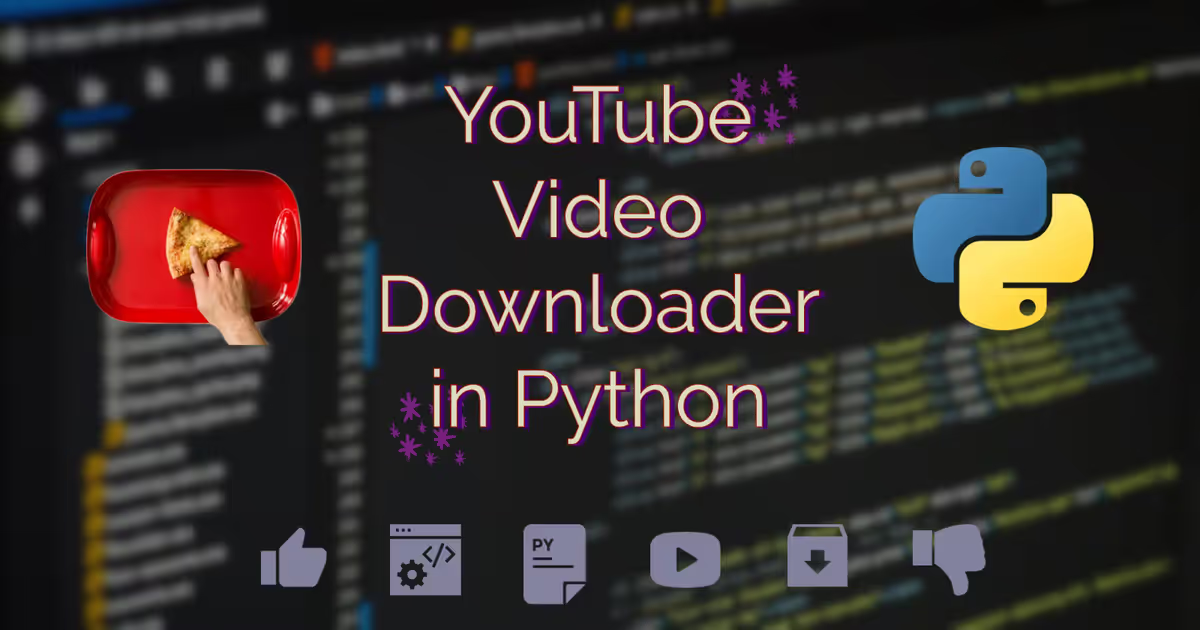
Learning a Programming language is a waste if you can't do cool things with it and things that makes your life easier.
Like you can control your appliances using microcontrollers like Arduino and program them to turn the light switch on/off with a single click on your phone.
These all are real life problems and you don't need propriety smart switches for that, you can make your own.
I think I'm getting a little ahead of myself and out of context for this article, though I'll surely discuss about microcontrollers and other cool stuff about SBC's very soon, so be prepared for that but right now we are going to write a script to download YouTube videos for us.
DISCLAIMER: Before I begin, let me make this very clear that I do support YouTube creators and I know they earn money when people subscribe to YouTube premium which lets users download videos on their devices and offscreen playback, etc. but let's be honest not everyone has money to pay for several subscription services and if you do have money then support your favorite creators/channels.
Okay enough moral talk, let's dive into the real thing. We're going to write a python script that will do the dirty work for us and for that we're using an awesome library which is called pytube.
Now if you don't know about Libraries, let me explain just a little bit about them, so a Library contains code that you can use and reuse to save time.
Like a real Library contains real books set accordingly to their genres. The same way a library works in python as well. Python comes preloaded with a standard library but you can always import more.
DISCLAIMER: This project is for education purpose only.
- I'm assuming that you've already installed python on your system and you have access to your favorite code editor. Now let's write some code.
- First you need to install the pytube library on your system and for that just write:
pip install pytube
- Okay, you have successfully installed the pytube library but you can't use it unless you import it in your script. For that, in your code editor, I'm using VS Code here, create a file, name it whatever you want with a
.py
extension. Like here I'm creating a new blank file named `yt-downloader.py`

- Now you have a python file to yourself, let's start by importing
pytube
library:
from pytube import YouTube
- Very well, now import
sys
library as well. Sys library is important here because we want user input via command line to our script.
import sys
- We want to input the URL of the video, so for that we are using a variable named
link
which we will later pass toYouTube
function
link=input("Enter the Video URL : ")
yt=YouTube(link)
- We don't want to blindly download anything from the URL we pasted, what if we accidently copied URL of some other video. For it not to happen, we are going to print some key elements of the video like the 'Title', 'Channel' , 'Length', etc. (Dashes are optional, I used them here for aesthetic purpose only .)
print("\n"+"------------------------------------------")
print("Title: ",yt.title)
print("Channel: ",yt.author)
print("Publish Date: ",yt.publish_date)
print("Views: ",yt.views)
print("Length: ",yt.length//60,"Minutes")
print("\n"+"------------------------------------------")
- Now we'll tell the script that we want to download the highest resolution available for that video, for that we are going to use
get_highest_resolution()
function.
yd=yt.streams.get_highest_resolution()
- We are almost done, I mean you can use the script right now and it will do the job for you but you might be thinking where is it going to save the video? We haven't provided the path yet. Well you can hardcode the path yourself right now or you can ask for user's input, which I'm gonna do here with some explanatory text.
filepath=input("Enter the path where you want to save, without quotations: "+'')
- If the user don't enter the path, the video will going to be saved in the same folder where the script is but if the user do enter the path like: in my case
/Users/Abhishek/Videos
it's going to be save in the videos folder of there system. - Now, I still haven't figured out how to show the download progress. So to overcome the suspicious feeling whether the download started or not, I'm adding this print statement to ensure the user that "Yeah, the download has REALLY started" and below that line, the real function that will download the video.
print("Download started in the background, do not close the program.")
yd.download(filepath)
- Last step, for users sanity, we are going to print the "Download Completed!" statement.
print("Download Completed!)
It's time to test our script now and to do that, open the terminal or command prompt whatever you're using and change the directory to where you have saved your python file, i.e., yt-downloader.py
file. I'm using VS Code's inbuilt Terminal feature which automatically takes me to the working directory.
Now to run the script, type:
python yt-downloader.py
And here you can see our script working, like asking the URL first:

Then printing out the relevant information we need:
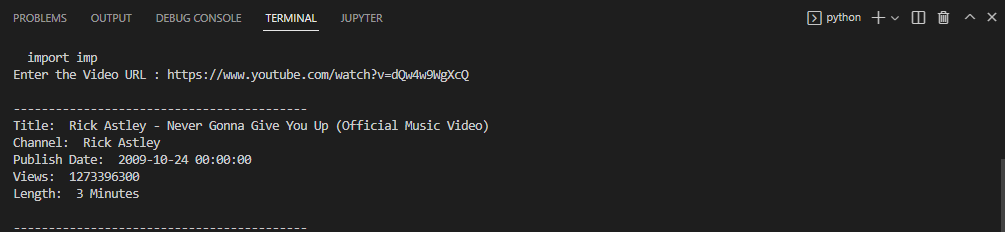
Asking for the path:
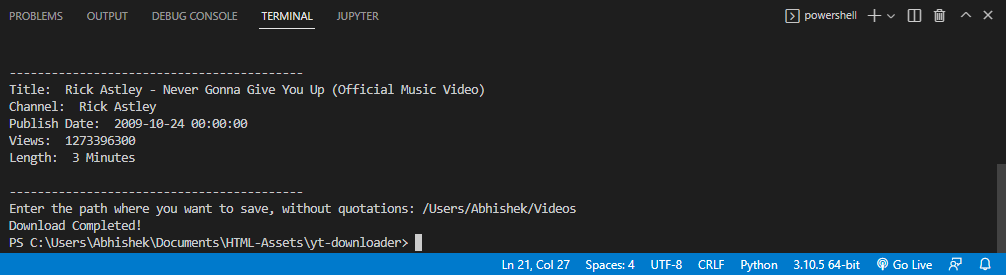
Printing out the "Download Completed!" popup at the end.
Now you can use this script to download any video you want from YouTube. You can even share this script with your friend but your friend have to setup the environment first before they can run this script. What if I tell you that you can turn your script into a standalone Windows executable script. I mean, you don't have to setup an environment and install bunch of libraries. What if you write it once and run on any Windows computer?
Let's take our script to another level by making it a Windows executable. Don't worry, it's just a two line piece of code that'll do our job. First install pyinstaller
library. You can read about pyinstaller's documentation for it's usage.
pip install pyinstaller
Now with the help of pyinstaller we can convert our python script to windows exe file. To use pyinstaller you have to be on the same directory where you have saved your script or you can just use path to locate your script but I'm in the same directory, so I'm just going to run this line of code:
pyinstaller --onefile yt-downloader.py
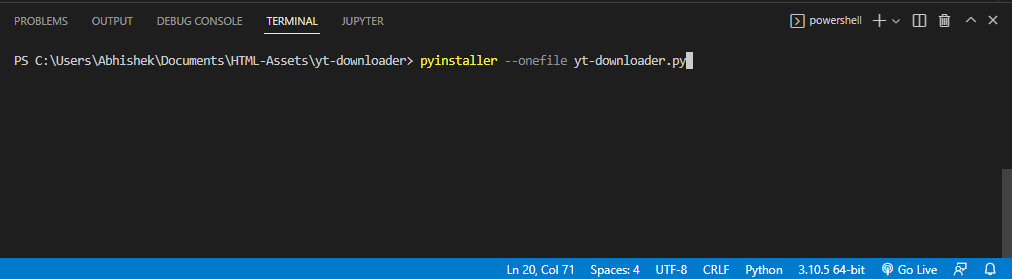
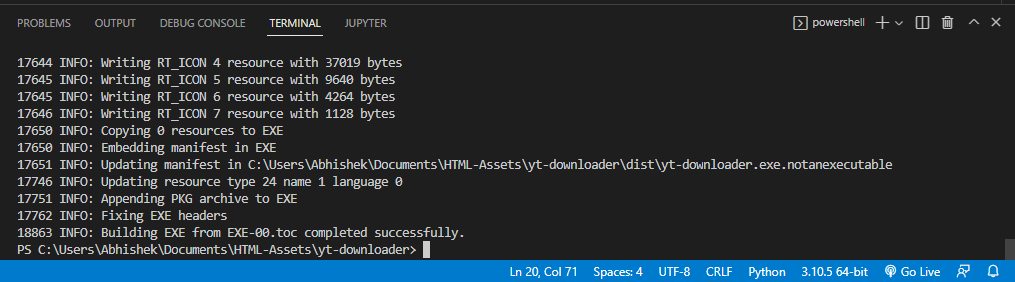
And it's done, you can now go to your directory where you have saved your python script and you'll see that there are bunch of other files and folders. You'll find your executable inside the dist
folder.
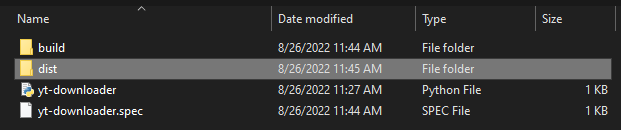
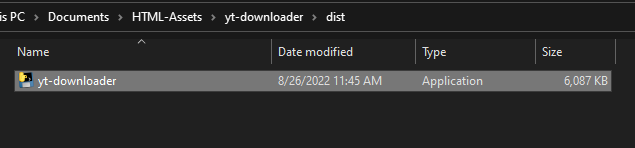
You can run this .exe on any windows computer and can even share with your less nerdy friends. If you still have any doubt, you can reference your code with mine, here's the link to my GitHub repository.
I think, I've covered this topic in the most easy and fun way and I hope you learned something cool with this tutorial.
If you have any doubt, you can always comment down below or reach me out on twitter.
Also, if you think that there's more efficient way to write this same code then do mention it, I've just started learning Python and I'm open to constructive criticism.